Automating TensorFlow Lite Builds with GitHub Actions for Raspberry Pi and x86
Automation is the backbone of modern software development, and GitHub Actions makes it easier than ever to build, test, and deploy software projects. When it comes to TensorFlow Lite, automating the build process ensures consistent, reliable results across platforms like Raspberry Pi (64-bit) and x86 (64-bit). This blog focuses on how GitHub Actions can simplify and streamline the CI/CD process for TensorFlow Lite builds, enabling developers to focus on innovation rather than manual setup.
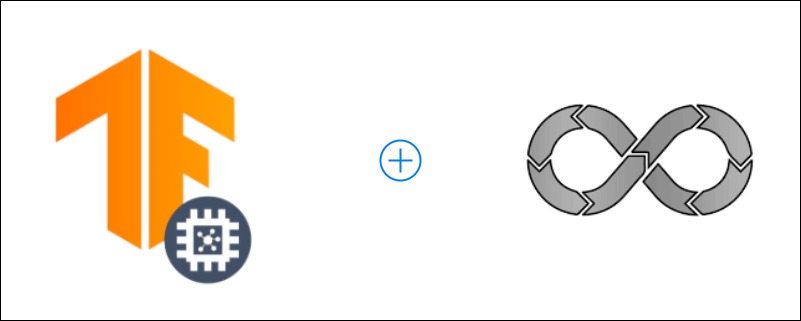
Why Use GitHub Actions for TensorFlow Lite?
You can find the complete implementation and source code in the GitHub repository: tflite-build.
Building TensorFlow Lite for specific platforms involves managing dependencies, configuring toolchains, and handling cross-compilation challenges. GitHub Actions automates this process, providing:
- Consistency: Ensures builds are repeatable and reliable.
- Efficiency: Automates time-consuming tasks like dependency installation and configuration.
- Scalability: Supports multiple architectures (Raspberry Pi 64-bit and x86).
- Accessibility: Produces precompiled
.deb
packages for easy deployment.
CI/CD Workflow Overview
Using GitHub Actions, the CI/CD pipeline includes the following steps:
- Cloning the TensorFlow Repository:
- Automates pulling the latest code from TensorFlow’s GitHub repository.
- Installing Dependencies:
- Handles installation of Bazel, Python, and necessary cross-compilation toolchains.
- Building TensorFlow Lite:
- Builds the TensorFlow Lite shared library for both Raspberry Pi and x86 architectures.
- Packaging into
.deb
Files:- Creates Debian packages for streamlined installation.
- Uploading Artifacts:
- Publishes precompiled packages to the GitHub Releases page.

Supported Platforms
Raspberry Pi
- Boards:
- Raspberry Pi 3 Model A+
- Raspberry Pi 3 Model B+
- Raspberry Pi 4 Model B
- OS:
- Raspberry Pi OS Bookworm 64-bit
x86
- Note:
- Does not support
avxnnint8
. This flag is disabled to ensure compatibility.
- Does not support
- Tested on:
- x86 64-bit machine running Ubuntu 22.04.
Installation
Raspberry Pi (64-bit)
Download and install the precompiled .deb
package:
wget https://github.com/<your-repo>/releases/latest/download/tensorflowlite-elinux_aarch64.deb
sudo apt install -y ./tensorflowlite-elinux_aarch64.deb
x86 (64-bit)
Download and install the precompiled .deb
package:
wget https://github.com/<your-repo>/releases/latest/download/tensorflowlite-amd64.deb
sudo apt install -y ./tensorflowlite-amd64.deb
Code Example: Using TensorFlow Lite
Here’s an example of how to use the TensorFlow Lite library in your C++ project:
#include "tensorflow/lite/interpreter.h"
#include "tensorflow/lite/kernels/register.h"
#include "tensorflow/lite/model.h"
int main() {
// Load the TensorFlow Lite model
auto model = tflite::FlatBufferModel::BuildFromFile("model.tflite");
if (!model) {
fprintf(stderr, "Failed to load model\n");
return -1;
}
// Build the interpreter
tflite::ops::builtin::BuiltinOpResolver resolver;
tflite::InterpreterBuilder(*model, resolver)(&interpreter);
if (!interpreter) {
fprintf(stderr, "Failed to construct interpreter\n");
return -1;
}
// Allocate tensor buffers
if (interpreter->AllocateTensors() != kTfLiteOk) {
fprintf(stderr, "Failed to allocate tensors\n");
return -1;
}
printf("TensorFlow Lite initialized successfully!\n");
return 0;
}
Benefits of Automation
- Ease of Deployment:
- Precompiled
.deb
packages simplify the installation process for end-users.
- Precompiled
- Reproducibility:
- Every build follows the same process, ensuring consistent results.
- Cross-Platform Compatibility:
- Supports both Raspberry Pi (64-bit) and x86 architectures.
Reference
With GitHub Actions, you can automate TensorFlow Lite builds for Raspberry Pi and x86 platforms, streamlining development and deployment processes. Try it out and simplify your workflows!
Enjoy Reading This Article?
Here are some more articles you might like to read next: